5. Calculating Booked Orders#
In this step of the workflow you will learn how n8n data is structured and how to add custom JavaScript code to perform calculations using the Function node.
The next step in Nathan's workflow is to calculate two values from the booked orders:
- The total number of booked orders
- The total value of all booked orders
To calculate data and add more functionality to your workflows you can use the Function node, which lets you write custom JavaScript code.
Function vs Function Item
n8n also provides a Function Item node, which should not be confused with the Function node. The Function Item node is used to add custom snippets of JavaScript code that should be executed once for every item that it receives as the input. Learn more about the difference between the Function and Function Item nodes here.
Before going into the setup of the Function node, you should first learn the data structure of n8n. This is important if you want to:
- Create your own node.
- Write custom expressions.
- Use the Function or Function Item node.
- Get the most out of n8n.
In n8n, the data that is passed between nodes is an array of objects with the following structure:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
|
Now let's see how to implement this.
In your workflow, add a Function node connected to the false branch of the IF node. In the Function node window paste the following code in the JavaScript Code box:
1 2 3 4 5 6 7 |
|
Notice the format in which we return the results of the calculation:
return [{json:{totalBooked, bookedSum}}]
Data structure error
If you don't use the correct data structure, you will get an error message: Error: Always an Array of items has to be returned!
Now execute the node and you should see the following results:
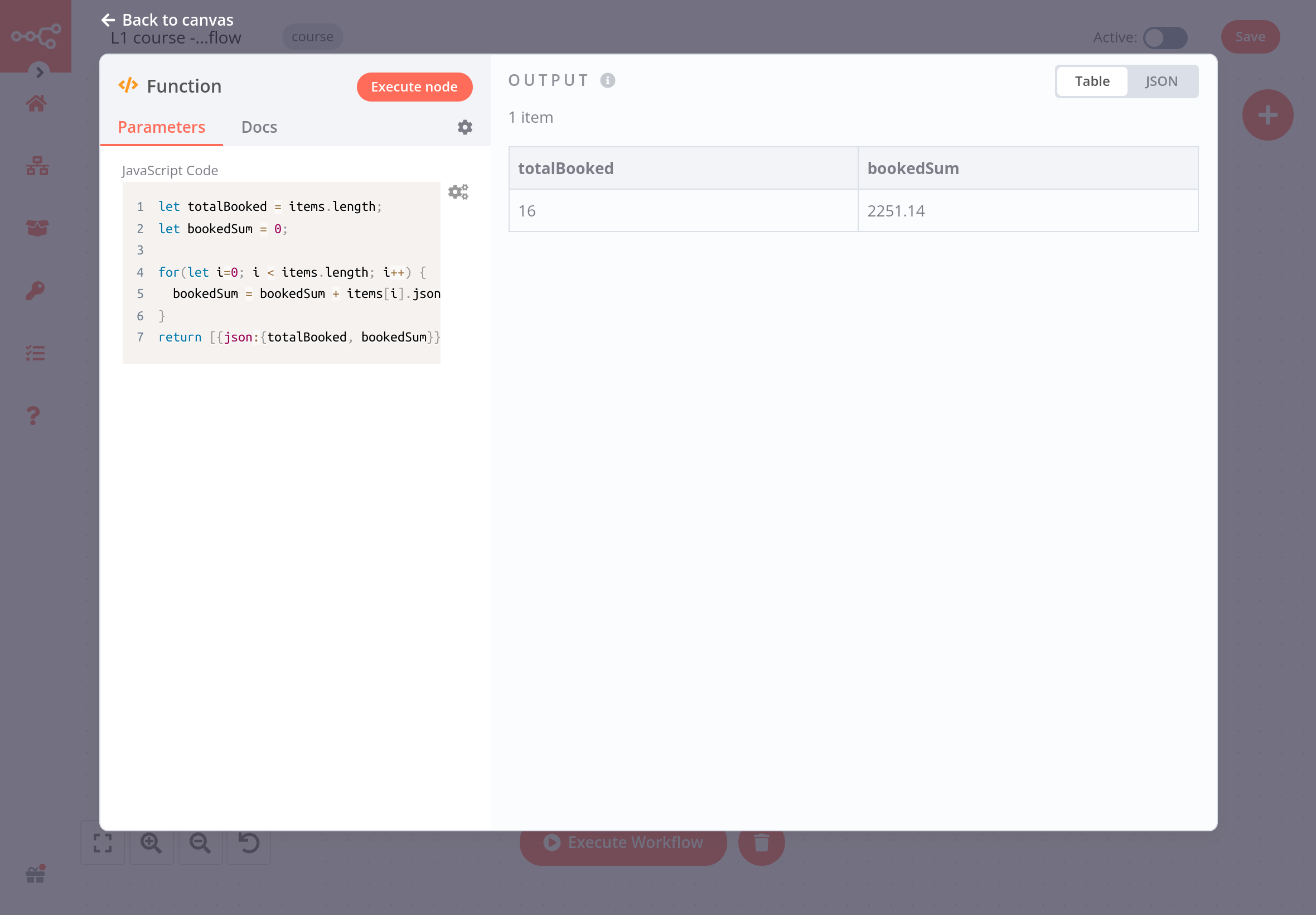
What's next?#
Nathan 🙋: Wow, the Function node is really powerful! So if I have some basic JavaScript skills I can power up my workflows.
You 👩🔧: Exactly – you can progress from no-code to low-code!
Nathan 🙋: Now, how do I send the calculations for the booked orders to my team's Discord channel?
You 👩🔧: There's an n8n node for that – I'll set it up in the next step.